Return
to IFS 110 class Notes Menu!
Project 3
Multiple Forms,
Dialogs, Debugging,
and EXEs
New Concepts
Project 3
- Dialog boxes - used
to ask for and supply information
- Debug Window - will be used
to find errors in the code
when program run
- Executable (stand-alone) applications -
run independently from
the software that was used to create them
- Visual Basics library
of built-in financial functions used
SavU Loan Analyzer
- Project calculates amount of monthly payment on a loan.
-
Icon consists of a
house and car.
- Inputs - amount of loan (uses
text box),
length in years, interest rate per year (uses scroll
bars)
Run-Time
Characteristics
Dialog box - used to display input error
(not a valid numeric amount)
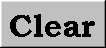 |
erases loan amount, monthly payment,
and sum of payments and returns scroll bars to
their lowest values |
|
displays a
dialog box
with icon, program
name, programmers name,
and date
|
SavU Loan Analyzer
System Menu
- Opened by clicking the System menu (Control-box) icon
- application can be minimized on desktop by clicking
Minimize on System menu or by clicking the
applications Minimize button
- Close application by choosing
Close on System menu or clicking
Close button

About...
Form Controls
Build the About...
form as follows:
- set the size of the form
- add the controls
- set the properties of the form and its controls
- save the form as a
file on diskette
Setting
Size of Form
Note: Code can be
used at run-time to change
the forms size by changing
the values (twips) of these properties
Image
Control
- Image
control can
be used as a container for
graphical images such as icons
or bit mapped graphics files.
(.bmp
extensions)
- Image control acts like a command
button,
so it often is used to create custom buttons like those
found in toolbars
Line
Control
Note: used to visually separate
parts of a
form (makes the user interface easier to understand)
Properties About...
Form
WindowState Property
WINDOWSTATE
VALUES
0-Normal
window open
on desktop 1-Minimized
window reduced to icon
2-Maximized
window set to maximum
size
|
Run-time
WindowState
changes
Note: You can control the ability to
make run-time changes to
the WindowState by including
or removing minimize
or maximize buttons from the form.
BorderStyle Property
Form
BorderStyle Property
Values
TABLE 3-2 VB 3.15
|
0-None1-Fixed Single
2-Sizable
3-Fixed Dialog
4-Fixed ToolWindow
5-Sizable ToolWindow
|
Note: If BorderStyle
is set to none , fixed-dialog, fixed ToolWindow, or
Sizable
ToolWindow the MinButton, MaxButton,
and ShowInTaskbar properties are set
automatically to False
Control Names
and Captions
Note: Important
to name forms, especially in projects
that contain more than one form.
Difference
between Name and
Caption
- Name - is the unique
identifier
of control or form used
by Visual Basic (cmdEnter) (Coding)
- Caption - is the label
attached to a control or form on the user
interface (Enter)
Font
Properties
- Font dialog box - used at
design-time
to change fonts,
font
size,
and font
style for text
and data fields (Table 3-3 VB 3.17)
- font characteristics
can be changed at run-time
using code statements
- Note: Font names available are
only those installed in Windows on
the current PC.
Picture Property
Image Control
Note: Visual Basic Package comes
with a set of icon files (.ICO)
(choose picture
property, double-click graphics subdirectory, choose icon subdirectory)
- .frx extension - form
containing
graphical
data is saved using this extension
Picture and
BorderStyle
Properties
Image Control
- If BorderStyle = 0 -
None -
size of image control adjusted to size of icon
- If BorderStyle
= 1 - Fixed single -
Visual Basic does not adjust its size
automatically
Note: Setting BorderStyle to Fixed
and the Stretch property to True will
cause the icon to be elongated to fill
the size of an image control
BorderStyle
Line Control
(Table
3-4 VB 3.20)
|
0 - Transparent
1 - Solid (default)
2 - Dash
3 - Dot
4 - Dash-Dot
5 - Dash-Dot-Dot
6 - Inside
Solid
BorderWidth
property - sets width of line
|
SavU Loan Analyzer
Form (steps)
- add a new form to project
- set the size of the form
- add the controls
- set the properties of the form
and
controls
- save the form
as a file on
diskette
Adding Shape
Controls
- Shape controls - used to
group related controls visually
- Inputs - data needed by
application to carry out
its function (input controls grouped within
shape control)
- Outputs - controls related to the
results
of the applications function
(output
controls grouped within shape control)
Coping
Controls
Keyboard Commands
- CTRL+C - copies selected
control to the Clipboard
- CTRL+V - pastes the control
from
the Clipboard to the form
Note: Important to set all the properties of the
control before the copy
so that all these properties can be copied
with the control
Adding Scroll
Bar Controls
- Scroll bars - used to
view
contents of a control when contents
cannot fit within the controls borders.
Note: another use of scroll bar is to give
a value to an input
advantage: prevents
you from entering an improper value by mistake.
(sets valid
range)
2 Kinds of
Scroll Bars
Scroll Bar
Change Event
- Change event is triggered
when you click a scroll arrow or drag
the scroll box
- Code statements can be written
linking
code to the change event that can change
the characteristics of other controls
tied to the scroll bar such as a caption of a
label
Setting
Properties
Alignment
Property
Text Boxes and Labels
- Specifies where caption will
appear will appear within the borders of a
control
- 0-Left Justify (default)
- 1-Right Justify (double-click)
- 2-Center
Note: to change text boxs
alignment, the value of
its MultiLine property must be equal to True (No longer true in VB6)
Scroll Bar
Properties
Scroll Bar
Properties
- SmallChange property
- amount
that the value changes each time you click one of the scroll
arrows
- LargeChange property
- amount
that the value changes each time you click the
area between
the scroll box and the two scroll arrows
Icon Property
of Forms
- Icon property - used
to specify the graphical
image (icon) used to represent the form
when it is minimized on the desktop
- Visual Basic contains a set
of icons (.ICO files) that
are in the COMMON\GRAPHICS\ICON
subdirectories
Writing Code
- Code written one event at a
time
- See TABLE 3-6 VB 3.42 for a summary of each of the LOAN PAYMENT APPLICATION
EVENT
PROCEDURES
Start Up Form
hsbYears_Change
and Scroll
Events
- Change event
triggered for
scroll bar any time the controls scroll
box is moved by clicking a scroll
arrow, dragging the scroll box,
or clicking the space
between the scroll box and scroll arrow.
- Scroll event - occurs when
scroll box dragged
- Movement of the scroll
box must be linked to a
new number displayed in the caption
of the label located above the scroll bar
lblYears.Caption
= hsbYears.Value
hsbRate_Change
and Scroll
Events
- Interest rate displayed
as the caption of lblRate must be converted
from value of hsbRate by multiplying it by .01
Note: This conversion
must be done in the code
rather than the properties setting section because the Max,
Min,
SmallChange and
LargeChange values can only be
set to integer (whole) values
Note: Code copied
from the Change to
Scroll
Event
lblRate.Caption =
hsbRate.Value * .01
cmdCalculate_Click
Subroutine
- Pmt function -
returns the payment for a loan based on periodic,
constant payments and a constant interest rate
- Function used in Code statement:
Pmt (rate,
nper,pv,fv,due)
- Arguments
- entries within the parentheses,
input values needed by the function
in order to return a value
PMT
FUNCTION ARGUMENTS
rate -
interest rate per period (monthly
= yearly rate / 12)
nper - total
number of payment periods (number
of years * 12)
pv - present
value is worth now to lender
fv - future
value or cash balance after
final payment (for loan future value
is 0)
due -
Number indicating when payments due (0
end of period, 1 beginning of
period)
|
Error
Trapping
- IsNumeric function: - will return a
True value if the contents are a number and a
False
value if the contents are not a valid number
- (FIGURE 3-77 VB 3.48)
Example:
IsNumeric (txtAmount.text)
If... Then
block
(FIGURE 3-78 VB 3.49)
Extension if Single-line If Statement
IF
condition THEN
statement1
statement2
ELSE
statement3
statement4
ENDIF
3 Parts of Message Box
- used to add dialog
box to application
MsgBox
text,type,title
- text body of message
box (enclosed in quotes)
- type number
represents type of button, type of icon,
whether dialog box is modal
- title text that
appears in title bar (enclosed in
quotes)
Online Help - combinations of buttons, icons, and
modality (Table
3-9 VB 3.49)
SetFocus
Method
Syntax:
controlname.Setfocus
cmdAbout_Click
Event
- modal - cannot
work with any other window until
the dialog box is closed
- forces user to respond
to dialog box
- modeless
- forms without this property
Note: you make forms visible on
the desktop and control their modality
at run-time
with the Show method in a
code statement
Form.Show
statement
form Name of
Form
to displaystyle
Integer value that determines
if form is
modal or modeless
(0 - modeless,
1 - modal)
Note: If style value not included (modeless)
Form_Load
Event
- Forms
Load
Event - causes code
statements to be carried out
when the form is loaded
into memory at run-time.
- Will use frmLoanabts Load
event
to assign captions of labels in the About
dialog box
Line
Continuation
- Line-continuation
character - combination
of a space followed by an underscore
( _) used in the development environment to extend
a single logical line of code
to two or more physical lines
- has no effect on execution
of code
- makes code much easier
to read and understand
- cannot use within a string
expression
- cannot continue one statement into more than 10 lines
Debugging
Applications
- Bug - error in
code
- Debugging - process of
removing errors
- Immediate window -
opens when you run program can be used to debug
program
- allows you to test
code statements without changing
any of your procedures
- Break button - temporarily
halt execution
of program (Standard
Toolbar)
- Continue button - resumes
execution
of halted program (Standard Toolbar)
Debugging
features
1. Viewing values
of variables and properties
(setting and viewing watch
expressions)
2. Halting execution
at a particular point
(setting a breakpoint)
3. Executing code one line
at
a time
(stepping through the code)
4. Executing code immediately
(the Immediate window)
Setting and
Viewing Watch Expressions
- Break mode - can isolate and
view values of particular variables
and properties
- Watch expressions - set to
specify which variables and properties
to monitor
- Immediate watch - used to
check the value
of any variable or property
in break mode
- Breakpoint - point at
which
you want to break execution
of program
Making Executable
Files
- To run an application outside of Visual Basic programming system, you must
compile, or convert, the
application into an executable,
or .exe, file
- Several advanced options
are available in the Project Properties window on the
Compile tab sheet
Project 3
Multiple Forms,
Dialogs, Debugging,
and EXEs
END
Program Future Value
Calculator Requirements
Return
to IFS 110 class Notes Menu!